【2DSTG制作】の動画内で表示されるコード:#51 – #56
サムネイルをクリックすると、YouTubeの動画ページに移動します。
#51:ボスユニットのギミック
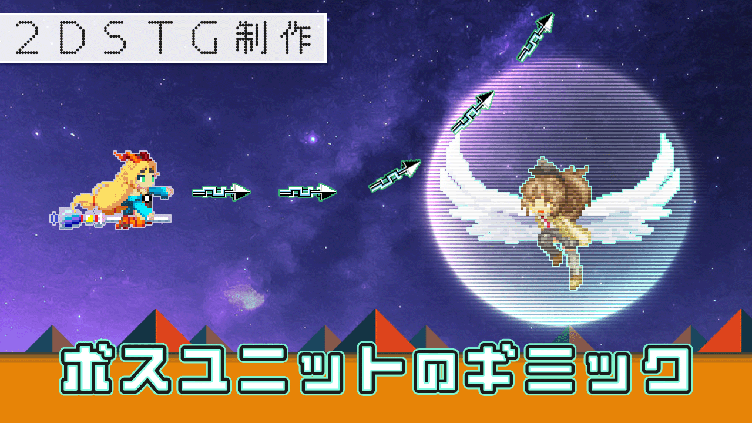
氷の設置
using UnityEngine;
using DG.Tweening;
// 停止する位置
[SerializeField] Vector2 targetPos;
// 爆発エフェクト
[SerializeField] GameObject explosion;
Rigidbody2D rb2d;
void Start()
{
rb2d = GetComponent<Rigidbody2D>();
var sequence = DOTween.Sequence();
// 指定した位置に移動する
sequence.Append(rb2d.DOMove(targetPos, 2.0f).SetEase(Ease.InQuart))
// 氷が存在する時間
.AppendInterval(10.0f)
.AppendCallback(() =>
{
// 爆発を生成して、氷を削除
Instantiate(explosion, transform.position, Quaternion.identity);
Destroy(gameObject);
});
}
#52:ゲームパッドでも遊べるようにしてみる
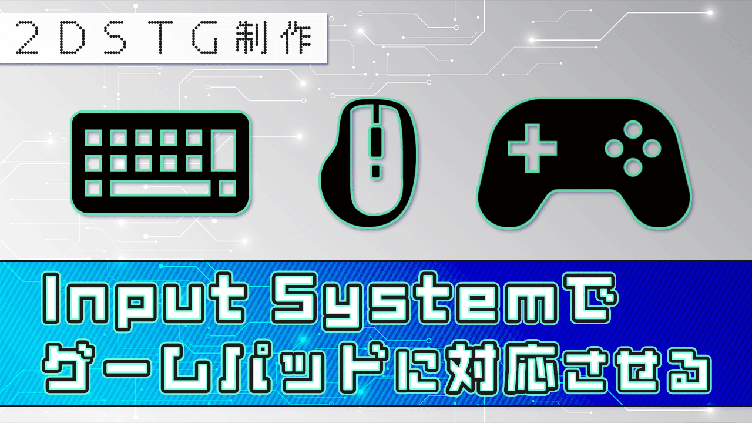
InputSystemでプレイヤーを操作
using UnityEngine;
using UnityEngine.InputSystem;
PlayerInput playerInput;
InputAction fire;
// 進む方向
Vector2 direction;
// 移動速度
float speed;
void Awake()
{
playerInput = GetComponent<PlayerInput>();
fire = playerInput.actions["Fire"];
}
void OnEnable()
{
playerInput.actions["Move"].performed += OnMove;
playerInput.actions["Move"].canceled += OnMoveStop;
playerInput.actions["Dodge"].performed += OnDodge;
playerInput.actions["Skill"].performed += OnSkill;
playerInput.actions["Style"].performed += OnStyle;
}
void OnDisable()
{
playerInput.actions["Move"].performed -= OnMove;
playerInput.actions["Move"].canceled -= OnMoveStop;
playerInput.actions["Dodge"].performed -= OnDodge;
playerInput.actions["Skill"].performed -= OnSkill;
playerInput.actions["Style"].performed -= OnStyle;
}
void OnMove(InputAction.CallbackContext context)
{
// 左スティックの値を取得
var value = context.ReadValue<Vector2>();
// プレイヤーの移動方向に設定
direction = new Vector2(value.x, value.y).normalized;
}
void OnMoveStop(InputAction.CallbackContext context)
{
// プレイヤーを停止
direction = Vector2.zero;
}
void OnDodge(InputAction.CallbackContext context)
{
// Dodgeのアクションに割り当てたキーが押されたら緊急回避
DodgeRoll();
}
void Update()
{
// OnMoveで取得した方向に移動
transform.position += direction * speed * Time.deltaTime;
// Fireのアクションに割り当てたキーが押されている間
if (fire.ReadValue<float>() > 0)
{
// ショットを撃つ
Shot();
}
}
void DodgeRoll()
{
// アニメーションを再生
animator.SetTrigger(dodgeParamHash);
// 入力に応じて移動
if (direction.x > 0.5f && direction.y > 0.5f) {
// 右上
transform.DOLocalMove(new Vector2(1, 1), 0.7f).SetRelative(true).SetEase(Ease.OutExpo);
// 右下に入力されていたら
} else if (direction.x > 0.5f && direction.y < -0.5f) {
// 右下
transform.DOLocalMove(new Vector2(1, -1), 0.7f).SetRelative(true).SetEase(Ease.OutExpo);
// 中略 //
} else if (Mathf.Abs(direction.x) < 0.2f && direction.y < 0) {
// X軸の入力が±0.2未満で、下方向に入力があれば下に移動
transform.DOLocalMove(new Vector2(0, -1), 0.7f).SetRelative(true).SetEase(Ease.OutExpo);
// デフォルトは右に移動
} else {
transform.DOLocalMove(new Vector2(1, 0), 0.7f).SetRelative(true).SetEase(Ease.OutExpo);
}
}
#54:入力に条件を設定してみる
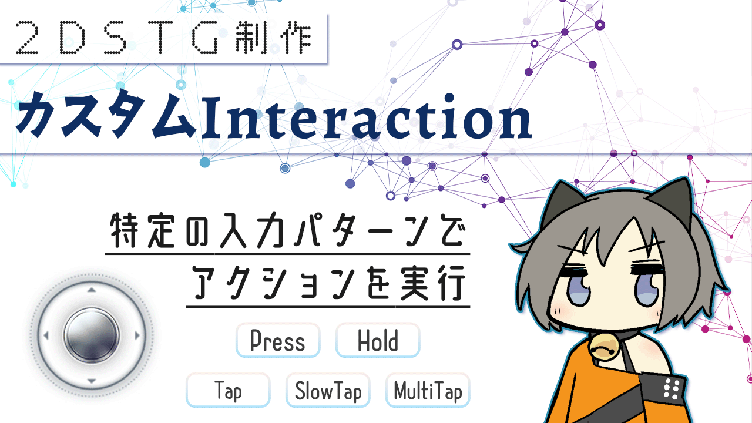
CustomInteraction
using UnityEngine;
using UnityEngine.InputSystem;
#if UNITY_EDITOR
using UnityEditor;
#endif
#if UNITY_EDITOR
[InitializeOnLoad]
#endif
public class WiggleInteraction : IInputInteraction
{
#if UNITY_EDITOR
static WiggleInteraction()
{
Initialize();
}
#endif
[RuntimeInitializeOnLoadMethod(RuntimeInitializeLoadType.BeforeSceneLoad)]
private static void Initialize()
{
// 作成したInteractionをInputSystemに登録
InputSystem.RegisterInteraction<WiggleInteraction>();
}
public float duration;
// ニュートラルポジションかどうか
private bool isNeutral = true;
// スティックを最初に倒した方向の確認
private bool rightStart;
public void Process(ref InputInteractionContext context)
{
// 左スティックの値を取得
var value = context.ReadValue<Vector2>();
if (context.timerHasExpired)
{
context.Canceled();
return;
}
switch (context.phase)
{
case InputActionPhase.Waiting:
// スティックの状態がニュートラルか確認
if (isNeutral == false)
{
if (Mathf.Abs(value.x) <= 0.1f)
{
isNeutral = true;
}
break;
}
// スティックを一定以上傾けた場合
if (Mathf.Abs(value.x) >= 0.8f)
{
// スティックを右側に倒した
if (value.x > 0) {
rightStart = true;
} else {
rightStart = false;
}
isNeutral = false;
context.Started();
context.SetTimeout(duration);
}
break;
case InputActionPhase.Started:
// 指定した時間内にスティックを反対側に動かすことができれば
if (value.x <= -0.8f && rightStart == true || value.x >= 0.8f && rightStart == false)
{
// Interactionが完了
context.Performed();
}
break;
}
}
public void Reset()
{
}
}